Firebase Cloud Messaging
Firebase Cloud Messaging (FCM) is a cross-platform messaging system that allows you to deliver messages with confidence. Utilize the Firebase Cloud Messaging (FCM) to send push notifications to users with android and enhance app re-engagement, as well as deliver data messages to your app.
You can send messages in 3 ways:
- To a single device.
- To a group of devices.
- To a group using subscribed topics.
You can send two types of messages:
- Notification Messages.
- Data Messages.
How to send push notifications on Android
First, I’ll teach you how to send push notifications without writing a single line of notification code using Firebase notification composer.
Create an Android project
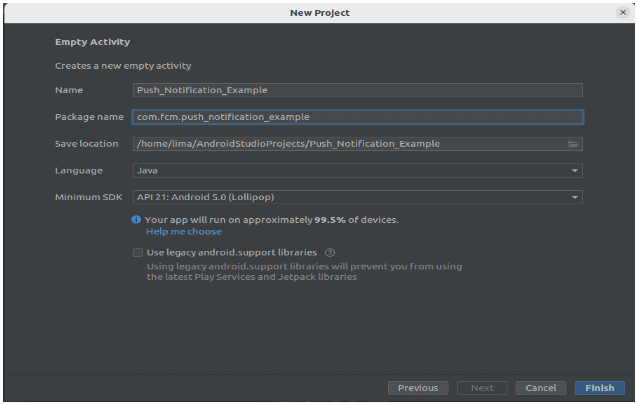
Then connect Android project with Firebase Tools -> Firebase
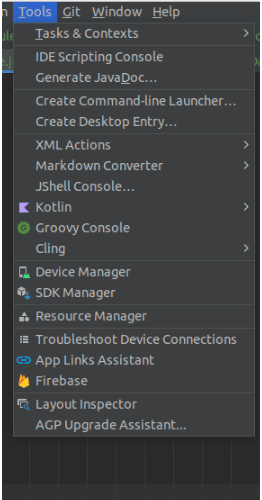
Click on Assistant and choose Cloud Messaging
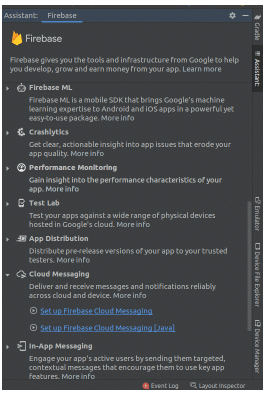
Click on Setup Firebase Cloud Messaging -> Connect to Firebase which will open the Firebase console and create a Firebase project. After creating the Firebase project it will automatically put the google-services.json to your Android project. Now click Add FCM to your app. After it is done go to Firebase Console and find Messaging.
Click on New Campaign and choose Notification
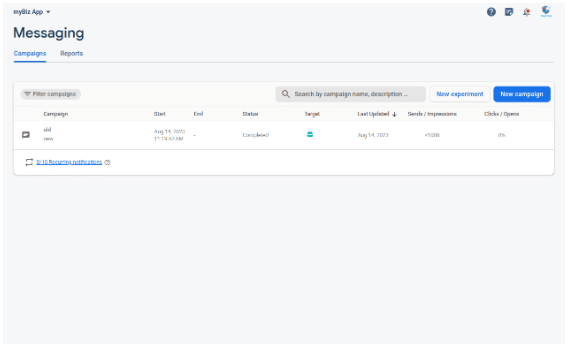
Fill in the details and click Next in Target you will target all apps.
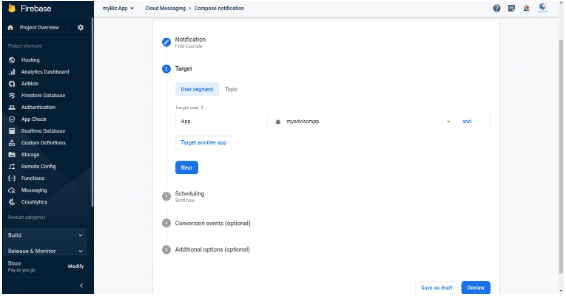
After filling in the details click Review then click Publish.
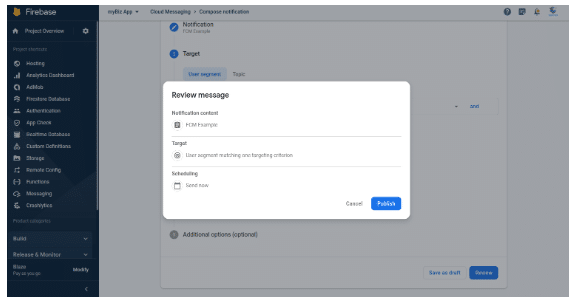
You will receive a notification like this
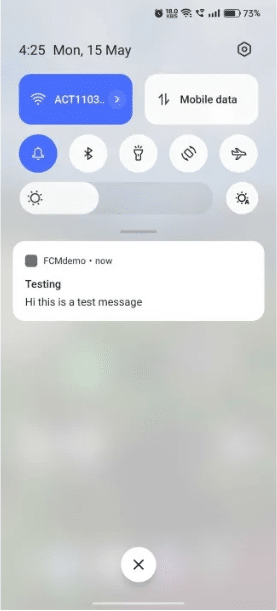
If no channel name is specified, the FSM SDK will establish a Miscellaneous notification channel.
Now, let’s handle notifications in the foreground. You need to extend FirebaseMessagingService.
class MyFirebaseMessagingService: FirebaseMessagingService() {
override fun onNewToken(token: String) {
super.onNewToken(token)
Log.i("FCM_TOKEN", token)
}
override fun onMessageReceived(message: RemoteMessage) {
super.onMessageReceived(message)
message.notification?.let {
val title = it.title
val body = it.body
val notification = NotificationCompat.Builder(this, App.FCM_CHANNEL_ID)
.setSmallIcon(R.drawable.ic_comment_24)
.setContentTitle(title)
.setContentText(body)
.build()
val manager = getSystemService(NOTIFICATION_SERVICE) as NotificationManager
manager.notify(1002, notification)
}
Log.i("DATA_MESSAGE", message.data.toString())
}
}
In onMessageReceived() you get both notification message and data.
Register this service to manifest.xml
<service
android:name=".MyFirebaseMessagingService"
android:exported="false">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT" />
</intent-filter>
</service>
Now, run the app in the foreground and compose a notification from Firebase. You should see the message in the foreground.
Make sure to create a notification channel for SDK Version >O
class App : Application() {
companion object {
const val FCM_CHANNEL_ID = "FCM_CHANNEL_ID"
}
override fun onCreate() {
super.onCreate()
if(android.os.Build.VERSION.SDK_INT >= android.os.Build.VERSION_CODES.O){
val fcmChannel = NotificationChannel(FCM_CHANNEL_ID, "FCM_Channel", NotificationManager.IMPORTANCE_HIGH)
val manager = getSystemService(NOTIFICATION_SERVICE) as NotificationManager
manager.createNotificationChannel(fcmChannel)
}
}
}
Remember to request POST_NOTIFICATION permission for Android 13 and higher.
<uses-permission android:name="android.permission.POST_NOTIFICATIONS"/>
// Declare the launcher at the top of your Activity/Fragment:
private val requestPermissionLauncher = registerForActivityResult(
ActivityResultContracts.RequestPermission(),
) { isGranted: Boolean ->
if (isGranted) {
// FCM SDK (and your app) can post notifications.
} else {
// TODO: Inform user that that your app will not show notifications.
}
}
private fun askNotificationPermission() {
// This is only necessary for API level >= 33 (TIRAMISU)
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.TIRAMISU) {
if (ContextCompat.checkSelfPermission(this, Manifest.permission.POST_NOTIFICATIONS) ==
PackageManager.PERMISSION_GRANTED
) {
// FCM SDK (and your app) can post notifications.
} else {
// Directly ask for the permission
requestPermissionLauncher.launch(Manifest.permission.POST_NOTIFICATIONS)
}
}
}
Once the permission is granted you can see the notification when you publish.
If you want to target a single device you need to have a registration token provided by FCM token.
FirebaseMessaging.getInstance().token.addOnCompleteListener(OnCompleteListener { task ->
if (!task.isSuccessful) {
Log.i("FCM_TOKEN", "Fetching FCM registration token failed", task.exception)
return@OnCompleteListener
}
// Get new FCM registration token
val token = task.result
// Log and toast
Log.d("FCM_TOKEN1", token)
Toast.makeText(baseContext, token, Toast.LENGTH_SHORT).show()
})
So that’s how you receive the token. If the user clears the app’s cache or uninstalls and reinstalls the app, you must override the onNewToken() method in FirebaseMessagingService.
class MyFirebaseMessagingService: FirebaseMessagingService() {
override fun onNewToken(token: String) {
super.onNewToken(token)
Log.i("FCM_TOKEN", token)
}
If you want to send a notification to a specific user, copy the token and paste it into Firebase notification composer, then click send test message, then paste the token and finally click send.
In conclusion, integrating push notifications into modern online and mobile apps through the usage of Firebase Cloud Messaging (FCM) is an effective strategy for user engagement and retention. Notifications to various devices may be sent more easily with the help of FCM’s stable and intuitive platform. FCM provides a dependable solution whether your goal is to improve user experience, increase user retention, or give real-time updates. Developers may improve the overall success of their apps by connecting with their audience and keeping them informed and involved by utilizing FCM’s capabilities and adhering to best practices.
Sreyas is a prominent software and mobile app development firm, boasting extensive expertise in UI/UX design. Our global presence allows us to offer a comprehensive range of services, including data migration, database management, web hosting, infrastructure management, and more to clients worldwide.