Register new Custom Post Type manually in WordPress.
In order to register a custom post type in WordPress, we must hook to the init action. The function.php file for the theme must contain the following code:
function prefix_create_custom_post_type() {
$args = array();
register_post_type( 'post-type-slug' , $args );
}
add_action( 'init', 'create_custom_post_type' );
The register_post_type() function, is a part of the prefix_create_custom_post_type() function. It is used to create a custom post type with parameters declared with $args.
Add parameters.
There are many different arguments that can be passed to $args, which makes things quite difficult. Therefore, be careful to be aware of what you require in order to select appropriate arguments. Only use some basic parameters and arguments to create a custom post type.
- The $labels are an insignificant parameter. But, it will help identify the post type in the admin section.
- The post type’s support for a title, editor, excerpt, featured picture, and other features is indicated by the $supports argument.
function prefix_create_custom_post_type() {
/*
* The $labels describes how the post type appears.
*/
$labels = array(
'name' => 'Products', // Plural name
'singular_name' => 'Product' // Singular name
);
/*
* The $supports parameter describes what the post type supports
*/
$supports = array(
'title', // Post title
'editor', // Post content
'excerpt', // Allows short description
'author', // Allows showing and choosing author
'thumbnail', // Allows feature images
'comments', // Enables comments
'trackbacks', // Supports trackbacks
'revisions', // Shows autosaved version of the posts
'custom-fields' // Supports by custom fields
);
/*
* The $args parameter holds important parameters for the custom post type
*/
$args = array(
'labels' => $labels,
'description' => 'Post type post product', // Description
'supports' => $supports,
'taxonomies' => array( 'category', 'post_tag' ), // Allowed taxonomies
'hierarchical' => false, // Allows hierarchical categorization, if set to false, the
Custom Post Type will behave like Post, else it will behave like Page
'public' => true, // Makes the post type public
'show_ui' => true, // Displays an interface for this post type
'show_in_menu' => true, // Displays in the Admin Menu (the left panel)
'show_in_nav_menus' => true, // Displays in Appearance -> Menus
'show_in_admin_bar' => true, // Displays in the black admin bar
'menu_position' => 5, // The position number in the left menu
'menu_icon' => true, // The URL for the icon used for this post type
'can_export' => true, // Allows content export using Tools -> Export
'has_archive' => true, // Enables post type archive (by month, date, or year)
'exclude_from_search' => false, // Excludes posts of this type in the front-end search result page if set to true, include them if set to false
'publicly_queryable' => true, // Allows queries to be performed on the front-end part if set to true
'capability_type' => 'post' // Allows read, edit, delete like “Post”
);
register_post_type('product', $args); //Create a post type with the slug is ‘product’ and arguments in $args.
}
add_action('init', 'prefix_create_custom_post_type');
Save the file. Then go back to the admin dashboard.
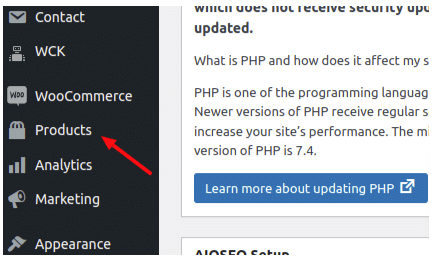
The following options will appear when your cursor is over this Product tab: All Products to display all posts of that Product type, Add New to create a fresh post in the Product category, etc.
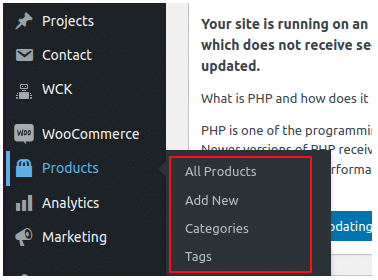
Now just finished the Custom post type using code.
For customizing your online presence, Sreyas IT is the best web and mobile app development company with a well-experienced staff. We are also a leading design company, that has hundreds of satisfied clients globally.