In cross-platform development, mapping weekdays or converting date representations between systems can be a common challenge. Today, we’ll look at a simple example where we convert weekdays in Dart to their corresponding representations in Java. This can be particularly useful for developers who build applications where code must interact between Flutter (using Dart) and Java-based backends or libraries.
Understanding Mapping Weekdays in Dart and Java
In Dart, DateTime weekday values start from 1 for Monday and end with 7 for Sunday. Java, however, starts with 1 for Sunday, followed by Monday as 2, and so forth. This difference means that if we want to match weekdays across Dart and Java, we need a mapping system.
Here’s how Dart and Java weekday values compare:
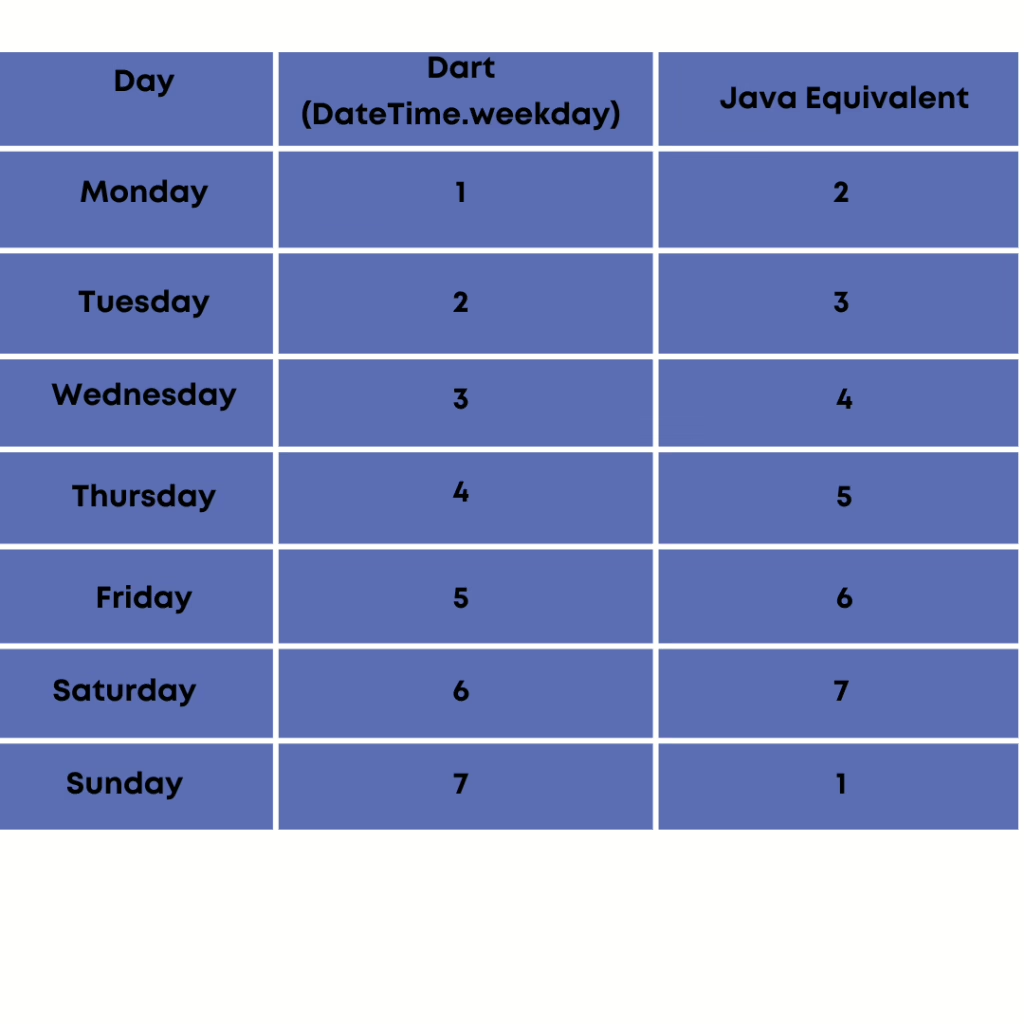
To create a seamless mapping between these representations, we’ll use a function that performs this transformation dynamically.
The Solution
We’ll create a Dart function called getWeek that takes a mode parameter. Depending on mode and certain conditions, this function will map Dart weekday values to their Java equivalents using a daysChange list. The code also checks the current time and day using DateTime.now() to determine whether the function should return a different mapped day.
Key Variables and Logic Breakdown
- daysChange Mapping Array: This array acts as a conversion lookup where each Dart day is mapped to its Java equivalent.
- Conditioning with mode: The function supports a mode parameter to switch between behaviors. When mode is 0, the function checks if a certain condition with time and date is met.
- Dynamic Date and Time: We use Dart’s DateTime.now() to pull in the current date and time.
Code Walkthrough
Let’s dive into the code.
int getWeek(int mode) {
// List to map Dart weekdays to Java weekdays
List<int> daysChange = [0, 2, 3, 4, 5, 6, 7, 1];
// Current date and time
DateTime current = DateTime.now();
int rh = 10; // Example threshold value; replace as needed
int hour = current.hour; // Current hour
int tz = 0; // Timezone offset; replace with your desired value
if (mode == 0) {
// If mode is 0, check if rh is greater than (hour + tz)
if (rh > (hour + tz)) {
return daysChange[current.weekday - 1];
}
}
// Default return if mode is not 0 or condition is not met
return daysChange[current.weekday];
}
void main() {
int mode = 0; // Example mode
int javaDay = getWeek(mode);
print("Converted day in Java format: $javaDay");
}
Code Explanation
- Mapping Array (daysChange):
- Each position in the daysChange list maps a Dart day to the equivalent Java day, with index adjustments for DateTime.weekday’s 1-based indexing.
- For example, daysChange[1] converts Monday in Dart to 2, the corresponding day in Java.
- Mode-Based Conditional:
- If mode is 0 and rh (a threshold value for this example) is greater than (hour + tz), the function returns the Java day for (current.weekday – 1).
- Otherwise, it defaults to returning daysChange[current.weekday].
- Dynamic Variables:
- The function uses current to pull the current weekday and time, making it flexible based on the actual date and time context.
Testing the Function
Run the code with mode = 0 and adjust rh, hour, and tz values as needed to see how it behaves under different conditions.
void main() {
// Testing with different modes and checking the output
print("Java day equivalent for mode 0: ${getWeek(0)}");
print("Java day equivalent for mode 1: ${getWeek(1)}");
}
Practical Applications
This function is ideal for scenarios such as:
- Cross-Platform Apps: If you’re building a Flutter app that communicates with a Java backend or server, aligning date representations can prevent misinterpretations of weekday data.
- Scheduling Apps: Apps that perform scheduling functions or calculate recurring events across multiple platforms benefit from using consistent weekday values.
- Localization: For apps that need to adapt weekday representations based on user settings, this function can help streamline conversions based on the active platform.
Conclusion
By implementing this Dart function, developers can seamlessly convert weekday values between Dart and Java formats. This simple solution highlights the importance of thoughtful date handling in cross-platform applications.